Connect with MQTT
Introduction
With the full system set up and the positioning working, we would like to extract the data out of the system to do something with it. This can be done very easily using the popular MQTT protocol. MQTT is an IoT protocol that allows to capture sensor data in a publish/subscribe method. By subscribing to a topic with the positions, you will get the newest positions as soon as they are available.
By default, only positions are embedded in the MQTT packet. However, it is possible to embed more sensor data from the tag in the packet. This can easily be configured in the settings page for positioning under settings > positioning. With the dropdown, it is possible to select all the different sensors.
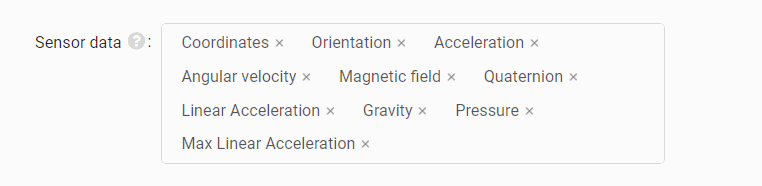
Connect with the local stream
When you need the data on your local device, you can connect directly when the Pozyx software is running (as can be seen in the tray application). Connecting locally is recommended for real-time applications as it provides a very low latency.
A lot of programming languages provide MQTT libraries. The following Python snippet subscribes to the local MQTT stream using paho-mqtt:
import paho.mqtt.client as mqtt
import ssl
host = "localhost"
port = 1883
topic = "tags"
def on_connect(client, userdata, flags, rc):
print(mqtt.connack_string(rc))
# callback triggered by a new Pozyx data packet
def on_message(client, userdata, msg):
print("Positioning update:", msg.payload.decode())
def on_subscribe(client, userdata, mid, granted_qos):
print("Subscribed to topic!")
client = mqtt.Client()
# set callbacks
client.on_connect = on_connect
client.on_message = on_message
client.on_subscribe = on_subscribe
client.connect(host, port=port)
client.subscribe(topic)
# works blocking, other, non-blocking, clients are available too.
client.loop_forever()
Connect through the cloud stream
By connecting through the cloud, you can receive your data anywhere, without requiring to be on the same device or the same network. The Pozyx cloud offers the MQTT data in a secure way. To gain access to the data of your setup, you must provide the correct credentials. These can be found in the application by going to Settings > API Keys. Here you can generate a secure key. Once you have created your key, you are ready to connect. By deleting a key, a consumer using that key will no longer be able to access to the data.
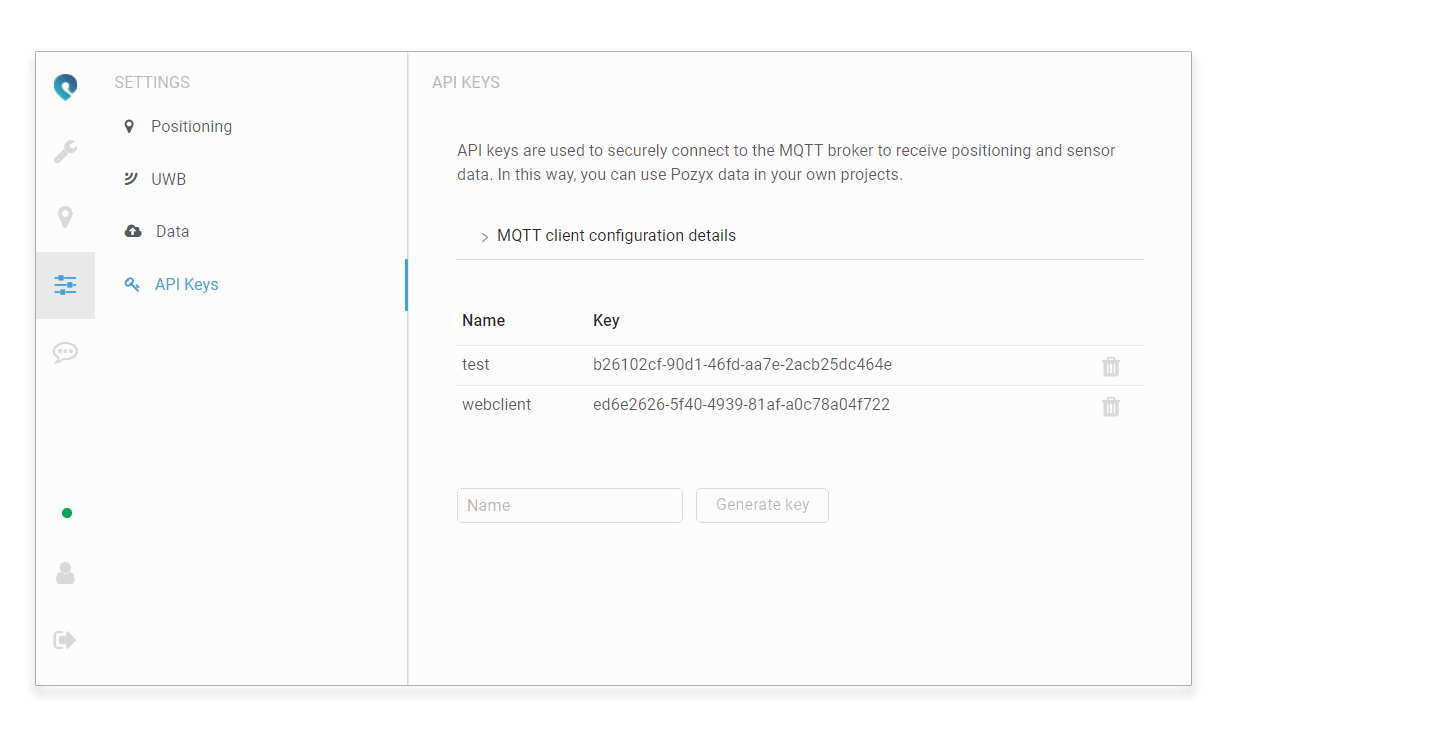
The MQTT service, provided as a WebSocket, is hosted on:
host = mqtt.cloud.pozyxlabs.com
port = 443
The remaining connection setting - topic, username, and password - can be found in the application by going to Settings > API Keys.
The following Python snippet subscribes to the cloud MQTT stream, using the credentials obtained as described above:
import paho.mqtt.client as mqtt
import ssl
host = "mqtt.cloud.pozyxlabs.com"
port = 443
topic = "" # your mqtt topic
username = "" # your mqtt username
password = "" # your generated api key
def on_connect(client, userdata, flags, rc):
print(mqtt.connack_string(rc))
# Callback triggered by a new Pozyx data packet
def on_message(client, userdata, msg):
print("Positioning update:", msg.payload.decode())
def on_subscribe(client, userdata, mid, granted_qos):
print("Subscribed to topic!")
client = mqtt.Client(transport="websockets")
client.username_pw_set(username, password=password)
# sets the secure context, enabling the WSS protocol
client.tls_set_context(context=ssl.create_default_context())
# set callbacks
client.on_connect = on_connect
client.on_message = on_message
client.on_subscribe = on_subscribe
client.connect(host, port=port)
client.subscribe(topic)
# works blocking, other, non-blocking, clients are available too.
client.loop_forever()
Please note that the cloud MQTT service caps the maximum update rate. This value can be set under settings. By default the update rate is capped to 10 Hz.
The MQTT data is sent as a JSON array. A single-packet-array with all sensor data enabled will look like this:
[{
{
"version": "1",
"tagId": "24576",
"success": true,
"timestamp": 1524496105.895,
"data": {
"tagData": {
"gyro": {
"x": 0,
"y": 0,
"z": 0
},
"magnetic": {
"x": 0,
"y": 0,
"z": 0
},
"quaternion": {
"x": 0,
"y": 0,
"z": 0,
"w": 0
},
"linearAcceleration": {
"x": 0,
"y": 0,
"z": 0
},
"pressure": 0,
"maxLinearAcceleration": 0
},
"anchorData": [],
"coordinates": {
"x": 1000,
"y": 1000,
"z": 0
},
"acceleration": {
"x": 0,
"y": 0,
"z": 0
},
"orientation": {
"yaw": 0,
"roll": 0,
"pitch": 0
},
"metrics": {
"latency": 2.1,
"rates": {
"update": 52.89,
"success": 52.89
}
}
}
}
}]