Logging data from the MQTT stream
If you want to log the positioning data for future analysis you can do this as follows:
Create a python script on your computer called logger.py:
import paho.mqtt.client as mqtt
import time
import sys
HOST = '<ip-address>'
PORT = 1883
TOPIC = 'tags'
DURATION = 300
def on_message(client, userdata, message):
print(message.payload.decode()) # 'utf-8'
def on_connect(client, userdata, flags, rc, properties):
print('Connected with result code ' + str(rc))
client = mqtt.Client(mqtt.CallbackAPIVersion.VERSION2) # create new instance
client.connect(HOST, port=PORT) # connect to host
client.on_connect = on_connect # attach function to callback
client.on_message = on_message # attach function to callback
client.loop_start() # start the loop
client.subscribe(TOPIC) # subscribe to topic
time.sleep(DURATION) # wait for duration seconds
client.disconnect() # disconnect
This script will print the positions for a duration of 5 minutes (300 seconds). Adjust the DURATION as needed, but don't make it too long because larger log files will be harder to process afterwards. Using the method described here we will create multiple smaller log files which you can always merge afterwards as needed.
Replace the IP address in the HOST variable with the IP address of your positioning server. You can find this by going to https://app.pozyx.io/devices/positioning-sever/info and searching for the Local IP in the Uplink network section:
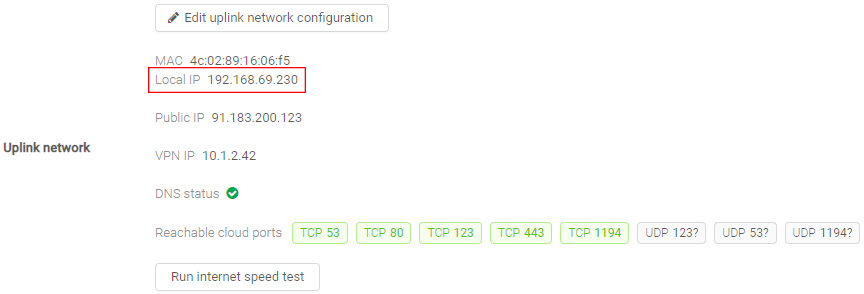
Next to the python script also create a shell script on your computer called logger.sh:
#!/bin/bash
for (( index=1; index<=$1; index++ ))
do
python3 logger.py > "$(printf "%01d" $index).log"
done
This script will execute the above python script and print the positions to a log file. A new log file will be created every 5 minutes, and it will do this a number of times (the exact amount of times will be specified later).
Make the shell script executable:
sudo chmod +x ./logger.sh
Execute the shell script:
./logger.sh 12 &
The first argument specifies the number of times you want to execute the python script. If you need to record for 1 hour and you have kept the DURATION in the python script at 300 then you will need to execute it 12 times.
The ampersand sends the execution of the shell script to the background (so you don't need to keep your terminal open after starting the script).